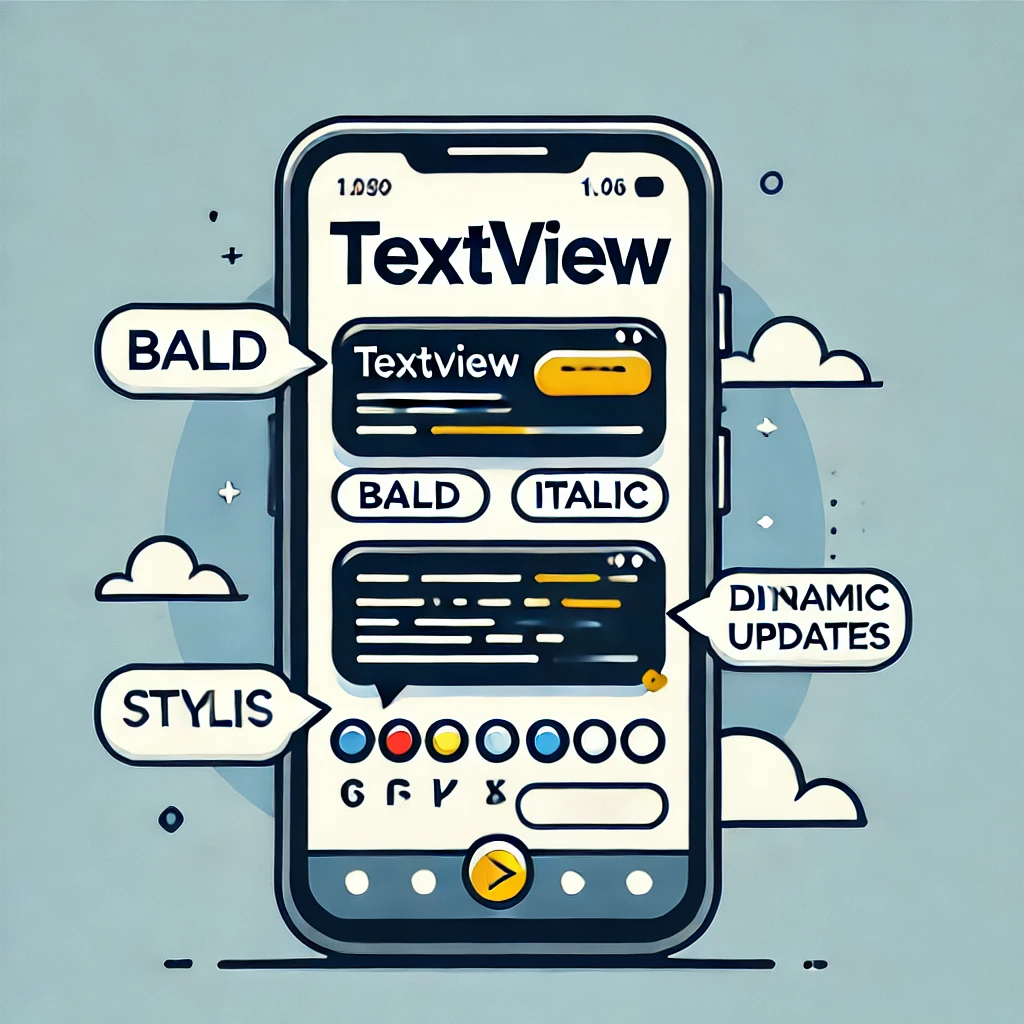
Introduction
TextView is one of the most fundamental and widely used widgets in Android development. If you need to show simple text, a heading, or content that changes based on your app’s logic, TextView is the best option. In this blog, we will explore the basics of TextView, its attributes, and how to use it effectively with coding examples.
What is TextView?
TextView is an Android widget that displays text to the user. It is part of Android’s UI and can show fixed and changing text. With its vast array of properties and methods, TextView can be styled, formatted, and customized according to your app’s needs.
Why Use TextView?
- Display Text: Ideal for showing text content like labels, instructions, or messages.
- Flexible Styling: Customize font, color, size, alignment, and more.
- Dynamic Content: Easily update text programmatically based on user interaction or backend data.
How to Use TextView in Android?
1. Basic TextView Example
Here’s how to create a basic TextView in an XML layout file:
<TextView
android:id="@+id/sampleTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!"
android:textSize="18sp"
android:textColor="#000000"
android:layout_margin="16dp" />
In this example:
text
sets the content of the TextView.textSize
adjusts the font size.textColor
changes the color of the text.
2. TextView with Custom Font and Alignment
Here’s how you can customize the font and alignment:
<TextView
android:id="@+id/customTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Welcome to Android Development!"
android:textSize="20sp"
android:textStyle="bold|italic"
android:gravity="center"
android:padding="10dp"
android:background="#FFDDFF" />
textStyle
adds bold or italic styling.gravity
arranges the text within the TextView (e.g., centre, left, right).background
sets a background color for the TextView.
3. Updating TextView Programmatically
You can update the text of a TextView dynamically in your Java or Kotlin code.
TextView textView = findViewById(R.id.sampleTextView);
textView.setText("Dynamic Text Updated!");
textView.setTextColor(Color.BLUE);
textView.setTextSize(22);
Best Practices for Using TextView
- Avoid Overloading: Don’t use too many styles or properties as it may reduce readability.
- Use Resource Files: Always use string resources (
res/values/strings.xml
) instead of manually writing the text for better localization and maintainability.
<string name="welcome_message">Welcome to Android!</string>
android:text="@string/welcome_message"
- Optimize Performance: Avoid using TextView for large text-heavy content; consider using ScrollView if necessary.
Advanced Features of TextView
Clickable Text:
<TextView
android:id="@+id/clickableTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me!"
android:clickable="true"
android:onClick="handleClick" />
public void handleClick(View view) {
Toast.makeText(this, "TextView Clicked!", Toast.LENGTH_SHORT).show();
}
Hyperlinks:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Visit Android.com"
android:autoLink="web" />
Multi-line Text: By default, TextView supports multi-line text. Use ellipsize
to manage overflow text.
android:ellipsize="end"
android:maxLines="2"
Conclusion
TextView is a powerful and versatile widget that plays a crucial role in Android app development. From displaying static labels to dynamic user messages, it provides the flexibility and tools needed to build intuitive UIs. With proper styling and best practices, TextView can enhance the appearance and functionality of your app.