Introduction
The Toolbar in Android is a versatile and powerful UI component that replaces the traditional ActionBar. It offers more customization and flexibility, enabling developers to create visually appealing and functional app headers. Whether you want to add navigation buttons, titles, menus, or logos, Toolbar provides everything you need to enhance your app’s design and user experience.
In this blog, we will dive into the basics of Toolbar, its customization options, and how to use it effectively in your Android apps with coding examples.
What is a Toolbar?
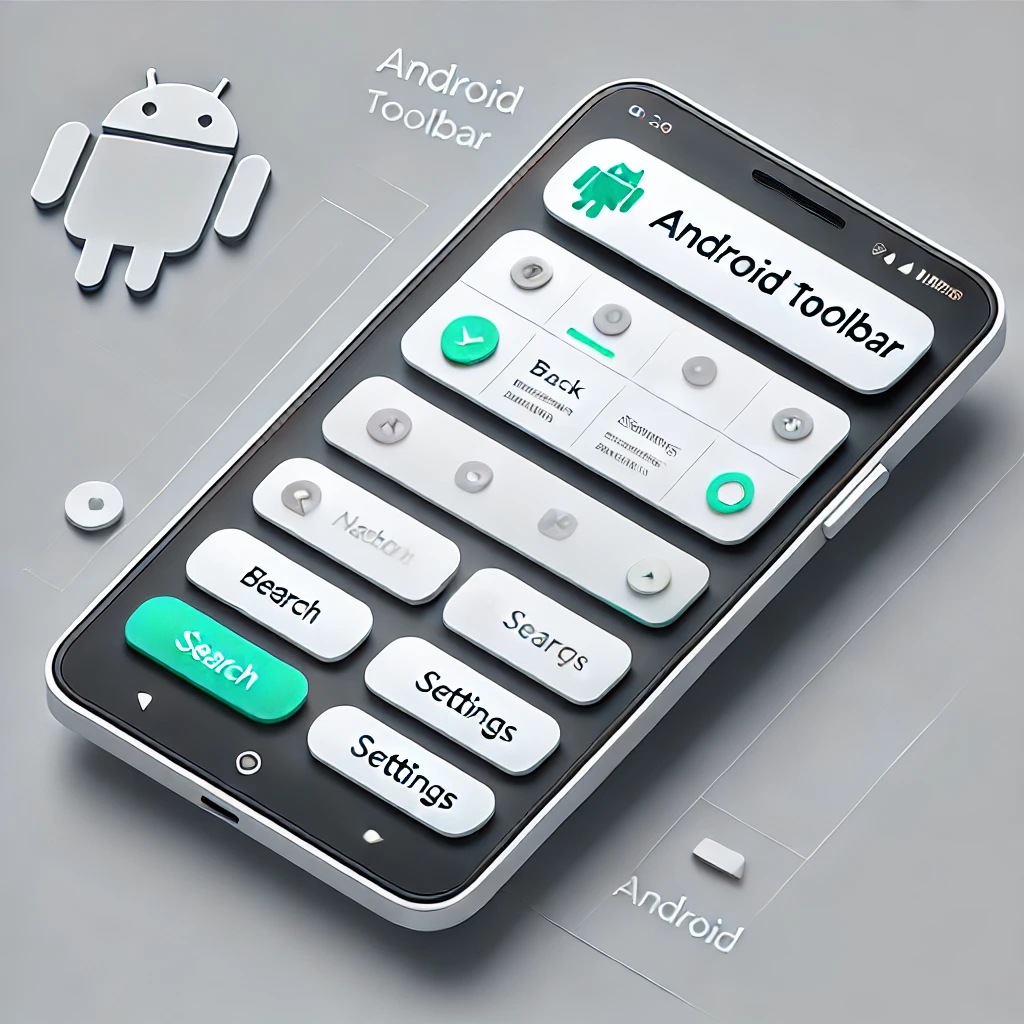
A Toolbar is a general-purpose container for app headers, replacing the ActionBar for more customization. It can display:
- App titles and subtitles.
- Navigation buttons (e.g., back or hamburger icons).
- Menu items and action buttons.
- Custom views, such as search bars or logos.
Why Use a Toolbar?
- Customizable: Offers flexibility to design app headers as needed.
- Interactive: Easily integrates navigation controls and menus.
- Modern Design: Aligns with material design principles for a polished look.
- Reusability: Can be used across multiple activities and fragments.
How to Use a Toolbar in Android?
1. Adding a Toolbar in XML
Here’s how you can add a basic Toolbar to your layout file:
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary"
android:elevation="4dp"
android:title="My Toolbar"
android:titleTextColor="#FFFFFF" />
Explanation:
background
: Sets the Toolbar’s background color.title
: Displays the title text.titleTextColor
: Specifies the color of the title text.elevation
: Adds shadow for a modern look.
2. Setting Toolbar in Activity
To make the Toolbar act as the app bar, use it in your activity:
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.Toolbar;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
getSupportActionBar().setTitle("Toolbar Example");
}
}
3. Adding Menu Items
You can add menu items to the Toolbar using a menu resource file:
menu_main.xml:
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/action_search"
android:title="Search"
android:icon="@drawable/ic_search"
android:showAsAction="ifRoom" />
<item
android:id="@+id/action_settings"
android:title="Settings"
android:showAsAction="never" />
</menu>
MainActivity.java:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
Customizing Toolbar
Here are some ways to style your Toolbar:
1.Add Navigation Icon:
toolbar.setNavigationIcon(R.drawable.ic_back);
toolbar.setNavigationOnClickListener(v -> onBackPressed());
2.Custom Fonts for Title:
Typeface customFont = Typeface.createFromAsset(getAssets(), "fonts/custom_font.ttf");
TextView toolbarTitle = toolbar.findViewById(R.id.toolbar_title);
toolbarTitle.setTypeface(customFont);
3.Add Logo:
toolbar.setLogo(R.drawable.ic_logo);
4.Adjust Toolbar Height:
android:layout_height="56dp"
Best Practices for Using Toolbar
- Consistency: Ensure the Toolbar is consistent across your app.
- Responsiveness: Use proper padding and alignment to adapt to different screen sizes.
- User-Friendly: Avoid overloading the Toolbar with too many items.
Conclusion
The Android Toolbar is an essential component for creating interactive and visually appealing app headers. It provides flexibility, ease of use, and a modern design aesthetic. By understanding its features and following best practices, you can enhance the user experience and make your app stand out.