Introduction
Creating engaging user experiences in Android apps often requires interactive UI elements. One such feature is the Android Popup, a small window that appears on top of the current screen to display important information or actions. Popups are versatile, user-friendly, and easy to implement, making them a popular choice for Android developers.
In this blog, we’ll explore what Android Popups are, their benefits, and how to implement them with examples.
What is an Android Popup?
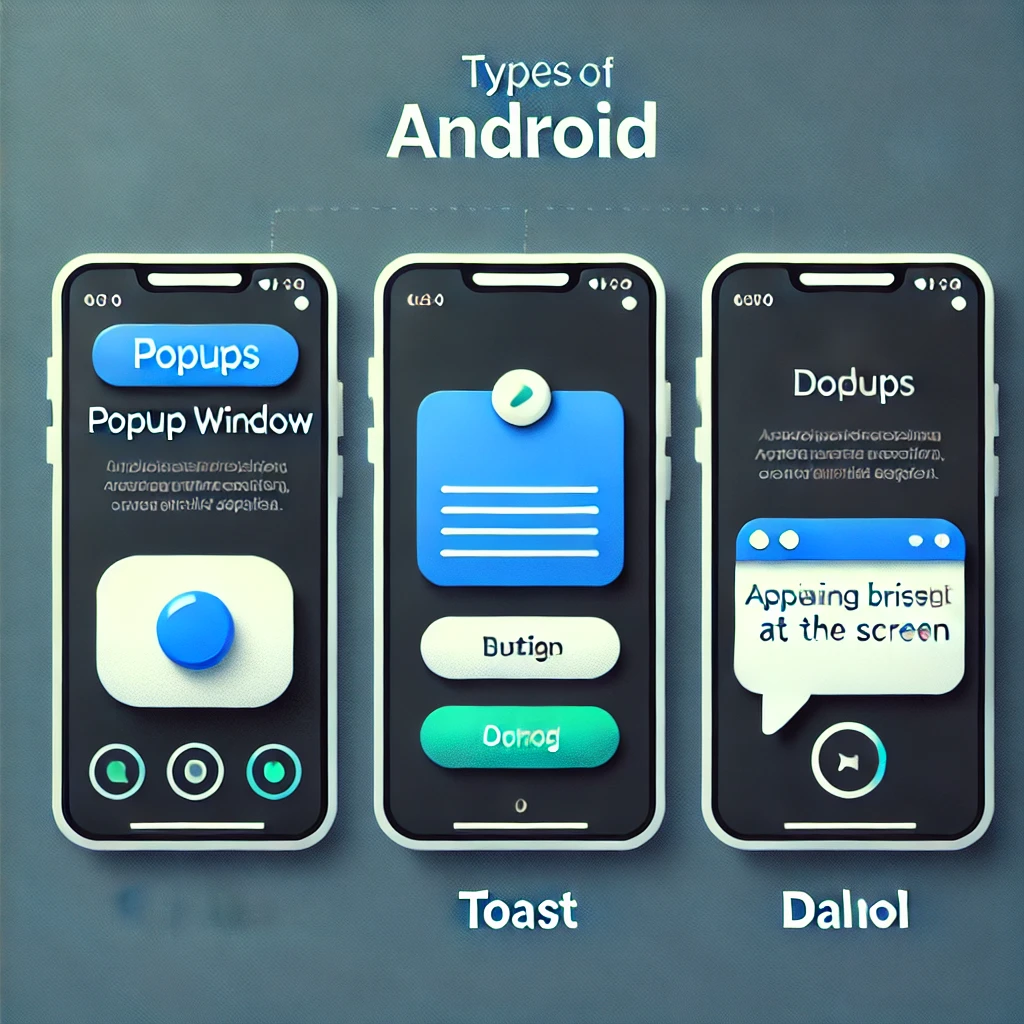
An Android Popup is a small, temporary window that appears over the current activity. It is used to show additional information, perform quick actions, or prompt users for input without navigating away from the main screen.
Types of Android Popups
1. Popup Window:
- A customizable popup that can include any UI elements.
- Ideal for displaying detailed information or interactive options.
2. Toast Message:
- A simple, non-blocking popup that appears briefly.
- Used for showing short messages, like “Action completed.”
3. Dialog:
- A popup that takes focus and requires user action.
- Commonly used for alerts, confirmations, or inputs.
Why Use Popups?
- Quick Information Display: Popups allow you to share important updates or actions without disrupting the app’s flow.
- Space Efficiency: They keep the main screen clean and uncluttered.
- Improved User Engagement: Interactive popups encourage users to take immediate action.
How to Implement an Android Popup
Let’s go through a step-by-step guide to create a Popup Window.
1. Popup Window Example
Step 1: Design the Popup Layout (popup_layout.xml)
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp"
android:background="@android:color/white">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is a Popup Window"
android:textSize="18sp"
android:paddingBottom="8dp" />
<Button
android:id="@+id/btnDismiss"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Close" />
</LinearLayout>
Step 2: Add Java Code to Show the Popup
public void showPopup(View view) {
LayoutInflater inflater = (LayoutInflater) getSystemService(LAYOUT_INFLATER_SERVICE);
View popupView = inflater.inflate(R.layout.popup_layout, null);
// Create the PopupWindow
PopupWindow popupWindow = new PopupWindow(popupView,
ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.WRAP_CONTENT,
true);
// Show the PopupWindow
popupWindow.showAtLocation(view, Gravity.CENTER, 0, 0);
// Close the Popup when the button is clicked
Button btnDismiss = popupView.findViewById(R.id.btnDismiss);
btnDismiss.setOnClickListener(v -> popupWindow.dismiss());
}
Step 3: Trigger the Popup in Your Layout
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Popup"
android:onClick="showPopup" />
2. Toast Example
A Toast is the simplest form of a popup in Android.
Toast.makeText(getApplicationContext(), "This is a Toast Message!", Toast.LENGTH_SHORT).show();
3. Dialog Example
A Dialog is another common type of popup. Here’s an example of an AlertDialog:
new AlertDialog.Builder(this)
.setTitle("Dialog Title")
.setMessage("This is a dialog message.")
.setPositiveButton("OK", (dialog, which) -> dialog.dismiss())
.show();
Best Practices for Using Popups
- Keep It Simple: Avoid overloading the popup with too much content.
- Focus on Relevance: Only use popups for essential information or actions.
- User-Friendly Design: Make the popup easy to dismiss and visually appealing.
Conclusion
Android Popups are an effective way to improve user interaction and display critical information. You can create engaging and user-friendly apps by understanding their types and proper implementation. Start incorporating Popups in your projects to take your app’s user experience to the next level!