Android Data Binding is a powerful library introduced by Google that allows you to bind UI components in your XML layouts directly to data sources in your application. It eliminates the need for boilerplate code, making your app development faster and cleaner.
What is Data Binding?
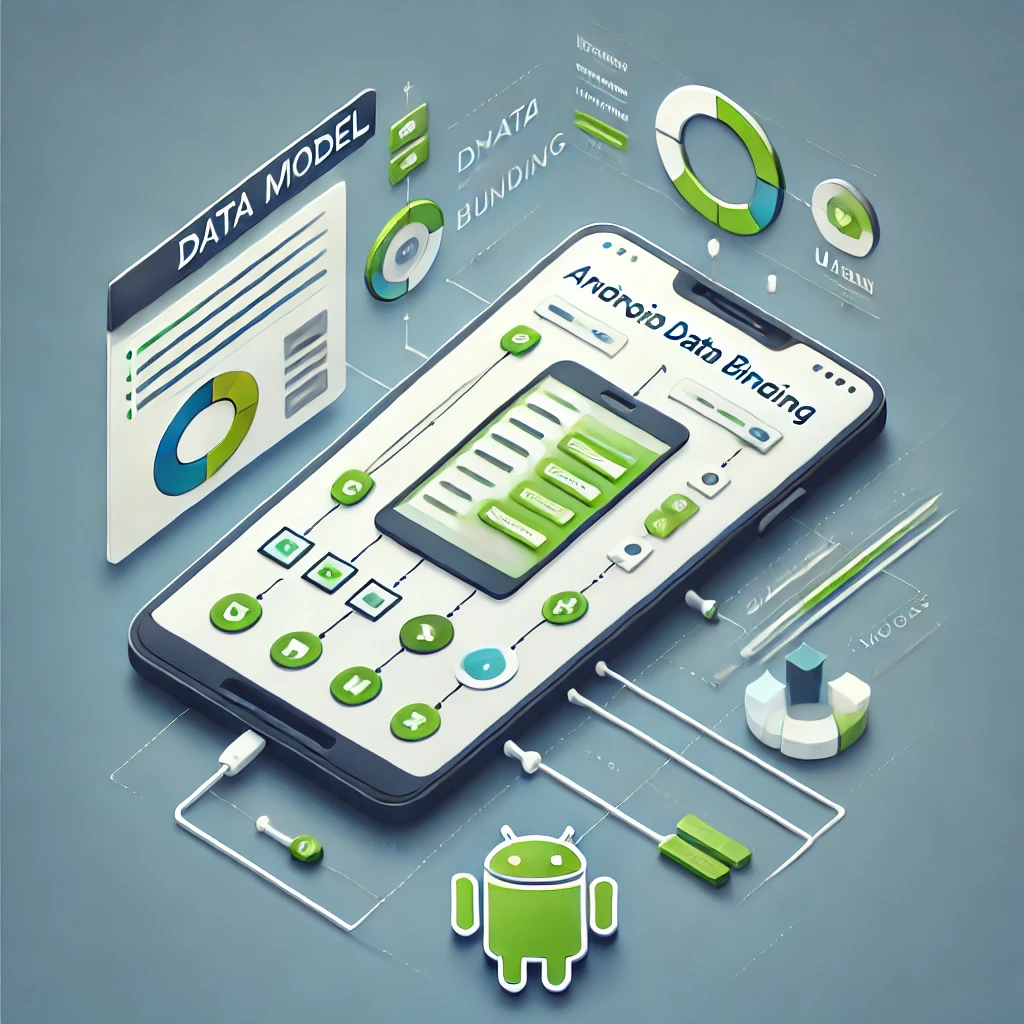
Data Binding is a feature of Android that connects the UI (User Interface) to the data model without requiring additional setup like findViewById() calls. It ensures a seamless connection, making your app more efficient and easier to maintain.
Why Use Data Binding?
- Eliminates Boilerplate Code: Reduces repetitive findViewById() and setter/getter methods.
- Enhanced Readability: Makes XML layouts more intuitive.
- Improved Performance: Minimizes memory leaks by using lifecycle-aware components.
- Two-Way Binding: Allows data changes to reflect in the UI and vice versa automatically.
How to Enable Data Binding?
To use Data Binding in your project:
- Enable it in the build.gradle file:
android {
...
buildFeatures {
dataBinding true
}
}
- Modify XML Layout: Wrap your layout in
<layout>
tags.
Example of Data Binding in Action
Here’s how to implement Data Binding step-by-step:
- XML Layout
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<data>
<variable
name="user"
type="com.example.app.User" />
</data>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{user.name}" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{user.email}" />
</LinearLayout>
</layout>
- Data Model
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
- Activity Class
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.databinding.DataBindingUtil;
import com.example.app.databinding.ActivityMainBinding;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ActivityMainBinding binding = DataBindingUtil.setContentView(this, R.layout.activity_main);
User user = new User("John Doe", "john.doe@example.com");
binding.setUser(user);
}
}
Two-Way Data Binding
Data Binding supports two-way binding to automatically update the data model and UI. For example:
- Add
@={}
in your XML:
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@={user.name}" />
- Ensure you use
BaseObservable
in your model:
import androidx.databinding.BaseObservable;
import androidx.databinding.Bindable;
public class User extends BaseObservable {
private String name;
@Bindable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
notifyPropertyChanged(BR.name);
}
}
Benefits of Using Data Binding
- Lifecycle Awareness: Automatically updates data when UI is active.
- Custom Binding Adapters: Extend functionality by defining custom attributes.
- Better Testability: Easier to test as logic remains in the ViewModel or Presenter.
Common Pitfalls and How to Avoid Them
- Null Pointer Exceptions: Always initialize your data objects.
- Complex Layouts: Avoid overloading XML with excessive logic.
Conclusion
Android Data Binding simplifies app development by directly connecting your UI components to your data. With reduced boilerplate code, improved performance, and advanced features like two-way binding, it’s a must-have tool for modern Android developers.
For more details visit: https://developer.android.com/topic/libraries/data-binding