Introduction to Fragments
In Android development, fragments are a powerful UI component that allows for more flexible app designs. To fully utilise fragments, it’s essential to understand their lifecycle.
A Fragment is a reusable portion of the user interface in Android. While an Activity is like a full screen or page in your app, a Fragment is a smaller part of that page. Fragments can be combined in one Activity, which makes them very useful for building flexible and dynamic user interfaces.
For example, in a tablet app, you might have a list of items on one side of the screen and a detailed view on the other. The list and the details are two separate Fragments within the same Activity. This allows developers to reuse and rearrange Fragments to create different layouts for different devices like phones or tablets.
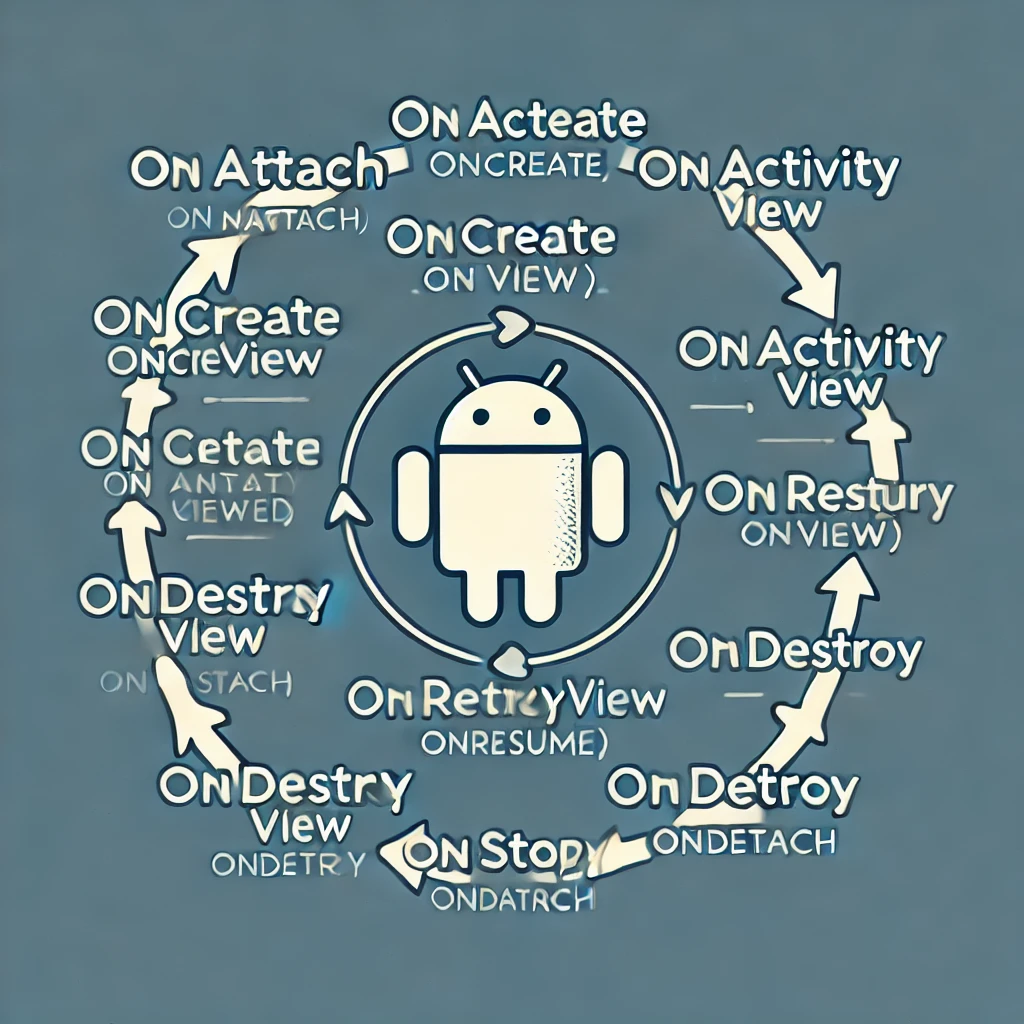
The Android Fragment Lifecycle
1. onAttach() – Fragment is Attached to Activity
When the Fragment is first attached to the Activity, onAttach()
is called. This is the stage where the Fragment gets connected to its parent Activity. Think of it as joining the two parts together, but the Fragment is not yet visible.
2. onCreate() – Fragment is Created
After being attached to the Activity, the onCreate()
method is called. In this phase, the Fragment is created and any essential setup is done, such as initializing data. It’s similar to how an Activity is created.
3. onCreateView() – Creating the Fragment’s UI
This is where the Fragment’s layout is created. The onCreateView()
method inflates the layout, which is the part of the screen that users see. It’s like setting up the visual part of the Fragment, such as buttons, text, and images.
4. onActivityCreated() – Activity is Ready
Once the Activity containing the Fragment has been fully created, onActivityCreated()
is called. Here, the Fragment can start interacting with the Activity, like sharing data or responding to user input.
5. onStart() – Fragment Becomes Visible
The Fragment is now visible to the user, but it’s not fully interactive yet. The onStart()
method makes sure the Fragment is in view, but the user can’t interact with it just yet.
6. onResume() – Fragment is Active
When the Fragment is ready for user interaction, onResume()
is called. Now, the user can interact with the buttons, text, or images in the Fragment. This is the stage when the Fragment is fully active.
7. onPause() – Fragment is Paused
If the user switches to another app or another screen, onPause()
is called. The Fragment is still visible but no longer interactive. It’s like taking a break, where the Fragment is still in memory but the user can’t interact with it.
8. onStop() – Fragment is Stopped
When the Fragment is no longer visible, onStop()
is called. It’s like the Fragment is hidden from view, but still in memory. The Fragment may stop some of its functions but can restart later.
9. onDestroyView() – Cleaning up the UI
The onDestroyView()
method is called when the Fragment’s view is no longer needed. This is where the visual part of the Fragment is cleaned up and removed from memory. The Fragment itself is still around, but its layout is destroyed.
10. onDestroy() – Fragment is Destroyed
When the Fragment is no longer needed, onDestroy()
is called. This is the stage where the Fragment is fully destroyed, and all its resources are released. It’s like closing a file after finishing your work.
11. onDetach() – Fragment is Detached from Activity
Finally, the onDetach()
method is called when the Fragment is completely disconnected from the Activity. This is the final stage, and the Fragment is no longer part of the app.
A Real-Life Example of the Fragment Lifecycle
Imagine you’re using a weather app with two sections: one shows the current weather, and the other shows the forecast.
- onAttach(): The forecast Fragment is attached to the main weather screen.
- onCreate(): The Fragment sets up the data for the weather forecast.
- onCreateView(): The Fragment’s UI is created and displays the forecast.
- onActivityCreated(): The Fragment starts interacting with the main screen.
- onStart(): The forecast Fragment becomes visible.
- onResume(): You can now scroll through the forecast.
- onPause(): You switch to another app, and the Fragment is paused.
- onStop(): The Fragment is no longer visible when you close the app.
- onDestroyView(): The UI for the forecast is removed.
- onDestroy(): The Fragment is destroyed as you close the app.
- onDetach(): The Fragment is completely disconnected from the main screen.
Why Does the Fragment Lifecycle Matter?
Fragments are an important part of modern Android apps. They help developers create flexible designs that work across different screen sizes. By understanding the Fragment lifecycle, developers can ensure that their apps run efficiently and handle changes, like screen rotations, without losing data or performance.
Managing Fragment State
Proper management of the fragment lifecycle is vital for maintaining the app’s performance and user experience. Understanding when to save and restore state data can prevent data loss.
In summary, the Android fragment lifecycle is crucial for managing how your app behaves. By comprehending these stages, you can create more dynamic and responsive applications that adapt to user interactions effectively.
To learn more about Fragments, visit the Android Developers documentation for in-depth explanations and best practices
Other Android components blog:
https://androidgurukula.com/comprehensive-android-tutorial-for-beginners/